Process Communication in Erlang
Hi there!
In erlang, no process talks directly to each other. You can think of it as a process in erlang has locked itself up in a room. And it is very workaholic. It loves doing its own work.
What about Concurrency and inter process communication?
Your one process can mail other process with required details and ask it to work on the data. (Erlang processes are workaholic). You just need the address of the process (the Process ID or the PID for this).
Lets take a very simple example, where in the erlang process prints the sound of the animal.
====================
-module(animal_sound).
-compile(export_all).
sound()->
receive
dog ->
io:format("bhuw bhuw");
cat ->
io:format("Meow");
_ ->
io:format("Whisperings!")
end.
====================
Save as animal_sound.erl, compile and run.
Note: compile(export_all) can be used when you want all your functions to be visible to the outside world. Else you can export only the visible functions like
-export([sound/0]).
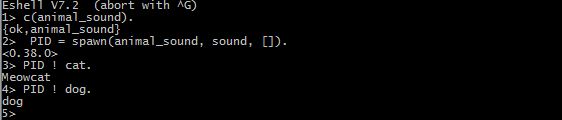
So why doesnot PID ! {self(), dog} print bhuw bhuwdog?
Let make a simple change in our animal_sound file and see the changes.
====================
-module(animal_sound).
-compile(export_all).
sound()->
receive
dog ->
io:format("bhuw bhuw"),
sound();
cat ->
io:format("Meow"),
sound();
_ ->
io:format("Whisperings!")
end.
====================
Again save, compile and run.

To understand the difference just run i(). command after ever command.

Here process ID (the PID) is <0.48.0>.
Run i(). You should be able to see a process like this.

i() prints all the processes running in the erl shell. Just search for your process.
Keep checking it after every command. In our 1st example -> after you call PID ! {self(), cat}, the process work is done and it stops. In the 2nd example -> after you run PID ! {self(), human}, the recursive call ends and i() does not show our Process ID. In other cases, the process is alive and ready to work!
Understanding inter-process communication:
There is a classic example of the Ping-Pong game to understand communication between two processes. Ping responds with a Pong and a Pong with a Ping. Lets take a look at it:
=====================
-module(pingpong).
-compile(export_all).
ping(0, Pong_PID) ->
Pong_PID ! finished,
io:format("Ping Finished ~n!", []);
ping(N, Pong_PID) ->
Pong_PID ! {ping, self()},
receive
pong ->
io:format("Ping received pong\n")
end,
ping(N-1, Pong_PID).
pong() ->
receive
finished ->
io:format("Pong finished\n");
{ping, Ping_PID} ->
io:format("Pong received\n"),
Ping_PID ! pong,
pong()
end.
start() ->
Pong_PID = spawn(?MODULE, pong, []),
spawn(?MODULE, ping, [4,Pong_PID]).
Since we don't want our ping pong game to run forever, we are giving a counter. After playing the game 4 times, the processes stop and the game is over.
Save as pingpong.erl. Compile and Run the example.
Done!
In erlang, no process talks directly to each other. You can think of it as a process in erlang has locked itself up in a room. And it is very workaholic. It loves doing its own work.
What about Concurrency and inter process communication?
Your one process can mail other process with required details and ask it to work on the data. (Erlang processes are workaholic). You just need the address of the process (the Process ID or the PID for this).
Lets take a very simple example, where in the erlang process prints the sound of the animal.
====================
-module(animal_sound).
-compile(export_all).
sound()->
receive
dog ->
io:format("bhuw bhuw");
cat ->
io:format("Meow");
_ ->
io:format("Whisperings!")
end.
====================
Save as animal_sound.erl, compile and run.
Note: compile(export_all) can be used when you want all your functions to be visible to the outside world. Else you can export only the visible functions like
-export([sound/0]).
So why doesnot PID ! {self(), dog} print bhuw bhuwdog?
Let make a simple change in our animal_sound file and see the changes.
====================
-module(animal_sound).
-compile(export_all).
sound()->
receive
dog ->
io:format("bhuw bhuw"),
sound();
cat ->
io:format("Meow"),
sound();
_ ->
io:format("Whisperings!")
end.
====================
Again save, compile and run.
To understand the difference just run i(). command after ever command.
Here process ID (the PID) is <0.48.0>.
Run i(). You should be able to see a process like this.
i() prints all the processes running in the erl shell. Just search for your process.
Keep checking it after every command. In our 1st example -> after you call PID ! {self(), cat}, the process work is done and it stops. In the 2nd example -> after you run PID ! {self(), human}, the recursive call ends and i() does not show our Process ID. In other cases, the process is alive and ready to work!
Understanding inter-process communication:
There is a classic example of the Ping-Pong game to understand communication between two processes. Ping responds with a Pong and a Pong with a Ping. Lets take a look at it:
=====================
-module(pingpong).
-compile(export_all).
ping(0, Pong_PID) ->
Pong_PID ! finished,
io:format("Ping Finished ~n!", []);
ping(N, Pong_PID) ->
Pong_PID ! {ping, self()},
receive
pong ->
io:format("Ping received pong\n")
end,
ping(N-1, Pong_PID).
pong() ->
receive
finished ->
io:format("Pong finished\n");
{ping, Ping_PID} ->
io:format("Pong received\n"),
Ping_PID ! pong,
pong()
end.
start() ->
Pong_PID = spawn(?MODULE, pong, []),
spawn(?MODULE, ping, [4,Pong_PID]).
=====================
Since we don't want our ping pong game to run forever, we are giving a counter. After playing the game 4 times, the processes stop and the game is over.
Save as pingpong.erl. Compile and Run the example.
Done!
Comments